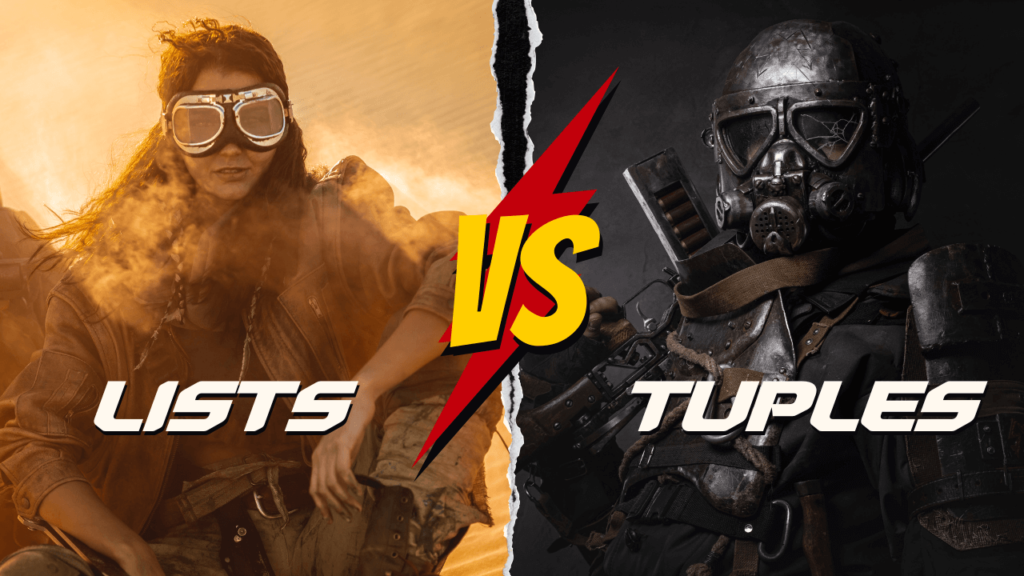
Do you know that one of the most commonly asked questions in Python technical interviews is the difference between tuples and lists?
The major difference between both data structures is that lists are mutable and dynamic while tuples are immutable and fixed. In other words, you can change the elements and length of a list and it’s impossible to change these properties with tuples.
However, that’s not all you need to know about the differences between Python tuples and lists.
This article will cover not only their similarities and differences but also show when to use Python List and Tuples for your projects.
What is a Tuple in Python?
A tuple is a built-in Python object that allows you to store ordered elements, separated by a comma and enclosed in parentheses ‘( )’. Tuples are immutable data structures. Once a tuple is created, it’s impossible to modify its value and length. This is why they are used to store data when data integrity is very important. Tuples can be used to store elements of different data types like numbers, strings, boolean values, null values, and other tuples.
Tuples support different operations like indexing, slicing, and iteration with a loop. You can also compare tuples and concatenate them with a ‘+’.
They are commonly used in scenarios where immutability and the order of elements matter, such as returning multiple values from a function, storing related data, or as keys in dictionaries. For example, tuples can be used to store map coordinates, or the x and y axis in 2D games – (x,y). Also, they can be used to store the day, month, and year (dd, mm, yyyy)
Here is an example of how to create a tuple:
User = ('Sam' , 23, 'Canada')
In this example, we can see that the User stores three elements with the string and number data types. To access the first element of the User tuple, we can use the User[0] and it will return ‘Sam’
Here are some examples of basic Python operations like slicing, indexing, etc:
point = (3, 5)
x = point[0] # Access the first element of the tuple
y = point[1] # Access the second element of the tuple
name, age, country = person # Unpacking the tuple into separate variables
slice_tuple = person[1:3] # Extract a portion of the tuple
length = len(person) # Get the length of the tuple
concatenated = person + point # Concatenate two tuplespython
What is a List in Python?
A list is a built-in Python data structure used to store ordered elements of various data types, separated by a comma and enclosed in a square bracket ‘[ ]’. Python List is mutable. This means their elements and sizes can be modified.
Lists are very versatile and popular in Python as they are used to store data whose values are bound to change over time. Lists are also used for storing sequences and implementing stacks and queues.
You can also perform operations like appending, indexing, extending, slicing, and many other operations with the list.
Here is an example of how to create a list in Python:
ages = [1, 2, 3, 4, 5]
In this example, a list ‘ages’ was created to store some numerical data types. You can access the values in the list by using the index value e.g. ages[0] would return the value 1.
Here are some examples of operations you can carry out with lists:
fruits = ["apple", "banana", "orange"]
fruits.append("kiwi") # Add an element to the end of the list
numbers = [1, 2, 3, 4, 5]
numbers[2] = 10 # Modify the element at index 2
names = ["Alice", "Bob", "Charlie"]
del names[1] # Remove the element at index 1
sliced = numbers[1:4] # Extract a portion of the list
length = len(numbers) # Get the length of the list
for item in fruits: # Iterate over the elements of the list
print(item)
Difference Between Tuples vs List
Python Tuples | Python List |
Tuples are immutable | Lists are mutable |
Insertions and deletions are easier with Python lists | List consumes more memory compared to tuples |
Tuples do not have many built-in functions | Insertions and deletions are easier with Python lists |
Iterations with tuples are relatively faster | Iteration with lists takes more time compared to python |
Tuples consume less memory | The list length can be modified and changed |
Tuples are enclosed in parenthesis | Lists are enclosed in square brackets |
Tuples length are fixed | List length can be modified and changed |
It’s important to note that because lists are mutable, care should be taken when sharing or modifying them, especially in scenarios where immutability is required or when dealing with large lists that may impact performance.
Python Tuple Vs List – Syntax Differences
One simple but powerful difference between a Python List and Tuple is their syntax. Tuples are enclosed in parenthesis () and the List is enclosed in square brackets.
Here is an example of how to create a list and tuple with Python:
my_list = [1, 2, 3, 4, 5]
my_tuple = (1, 2, 3, 4, 5)
print(type(my_list))
print(type(my_tuple))
Output:
<class 'list'>
<class 'tuple'>
Mutability – Mutable Tuple vs Mutable List
We have heard about mutability which simply means the ability to change the length and element of a data structure. In Python, tuples are immutable and lists are mutable. Let’s explain this concept with some examples.
my_list = [1, 2, 3, 4, 5]
# Let's try changing the first element of the list
my_list[0] = 9
my_list
Output:
[9, 2, 3, 4, 5] |
On the other hand, if we try to change the value of an element in a tuple, we are faced with an error. Look at the example below.
>>> my_tup (1, 2, 4) >>> my_tup[2] = 5 Traceback (most recent call last): File “<stdin>”, line 1, in <module> TypeError: ‘tuple’ object does not support item assignment |
Python List Vs Tuple – Size and Memory Comparison
Due to the dynamic nature of a list, it tends to consume more memory than a tuple. You might not notice the difference between the two with small sizes. But as the size of the tuple and list increases, you notice that List uses more memory than tuples.
Here is an example to back up our claims:
my_tup= (1,2,3,4,5,6,7,8,9,0)
my_list= [1,2,3,4,5,6,7,8,9,0]
print(my_list.__sizeof__())
print(my_tup.__sizeof__())
Output:
104
120
Similarities Between Tuples and List
Although tuples and list have their differences. However, they share a lot in common than one could imagine. Here are 5 similarities between Tuples and Lists in Python.
- They are both used to store data.
- They can store data of various data types.
- Python Tuple and List are ordered. This means they can retain the order of their elements
- Elements of tuples and lists can be accessed by an integer index operator, given in square brackets, [index].
- Python Liss and Tuupele are sequential data. This means that you can iterate over their elements.
Common Python List Operations
Here are a list of some available operations you will likely use in your everyday work with pythons. You should bookmark this page so you can always make reference to it when needed.
How To Append or Add To A List In Python – append()
To add an element to the end of a list. You use the append command. The append command adds just one element at a time. Here is an example:
>>> my_list
[9, 2, 3, 4, 5]
>>> my_list.append(6)
>>> my_list.append(7)
>>> print(my_list)
[9, 2, 3, 4, 5, 6, 7]
How To Sort A List In Python – sort()
To sort a list in Python in ascending order. You use the `.sort()` command. You can only perform this operation when the list contains elements with the same data type. Heterogenous data will throw an error.
>>> my_list
[9, 2, 3, 4, 5, 6, 7]
>>> my_list.sort()
>>> print (my_list)
[2, 3, 4, 5, 6, 7, 9]
How To Reverse A List In Python – reverse()
To reverse an original list by rearranging the elements from the back. You use the `.revers()` command. However, if you do not have the intention of reversing the original list but want to use the reversed order for any operation. You can use slicing to reverse the string.
>>> my_list
[2, 3, 4, 5, 6, 7, 9]
>>> my_list2 = my_list.copy()
>>> my_list2
[2, 3, 4, 5, 6, 7, 9]
>>> my_list.reverse() #using the .reverse() method
>>> my_list
[9, 7, 6, 5, 4, 3, 2]
>>> my_list2[::-1] # reverse the list by slicing
[9, 7, 6, 5, 4, 3, 2]
How To Get Length Of A List In Python – len()
To get the length of a list, you can use the len() method and add the list as an argument.
>>> my_list
[2, 3, 4, 5, 6, 7, 9]
>>> len(my_list)
7
How To Remove An Item From A List In Python – remove()
The remove method is used to delete the first occurrence of an item from a list. If there are multiple elements, the remove method ignores other occurrences as shown in the example below.
>>> some_list = [2, 3, 4, 2, 5]
>>> some_list.remove(2)
>>> print(some_list)
[3, 4, 2, 5]
How To Copy A List In Python – copy()
There are several ways to create a copy of a list with Python. However, you can use the copy() method to create a shallow copy.
prime_numbers = [2, 3, 5]
# copying a list
numbers = prime_numbers.copy()
print('Copied List:', numbers)
# Output: Copied List: [2, 3, 5]
How To Insert Element Into A List – insert()
The insert() method allows you to add a new element to a list in a given position. Unlike append, the insert does not add elements at the end. It takes two arguments, the first argument is the new item position, and the second argument is the element value. You can only insert one element at once. Hence for inserting multiple elements, you can use a loop.
some_list = [1,2,3]
some_list.insert(3, 4)
some_list.insert(4, 5)
some_list.insert(5, 6)
print(some_list)
When To Use Tuple Over List
Tuples and lists are both used in Python to store collections of data, however, they differ in certain key ways. Here are some scenarios where tuples would be preferable to lists:
Immutable Data – Because tuples are immutable, you cannot modify its elements after they are formed. Tuples are thus a good choice for storing data that change, including setup parameters, constant values, or other data that should remain constant while your program is running.
Performance – Because tuples are immutable and lighter, they may be quicker to generate, access, and iterate through than lists. If you need to store, retrieve, and use a large amount of data often. And you do not need to amend the data, using a tuple may be more efficient than using a list.
Data Integrity – Tuples can be used to assure data integrity by ensuring that the data’s structure and contents remain consistent. If a function returns a certain number of values, for example, you might want to deliver them as a tuple rather than a list so the caller is aware of how much data to expect.
Conclusion
Now that you’ve understood the difference between Python list and Python tuples. The major difference between tuples and lists in Python is dependent on their mutability. Tuples are immutable while lists are mutable. Now that you’ve grasped this concept. You can apply it in your project or give the right answer when asked in an interview.
However, I will not advise you to stop there.
Are you interested in boosting your understanding of Python data structures? Check out our article on the difference between a dictionary and a list.